Hi Coders! Welcome to our new article on inheritance in C++ Questions and Answers. In this article, we will delve into the fundamental concepts of inheritance, types of Inheritance, benefits, and various related aspects. Whether you're a beginner or looking to strengthen your understanding of C++ inheritance, this article is for you. So, let's get started!
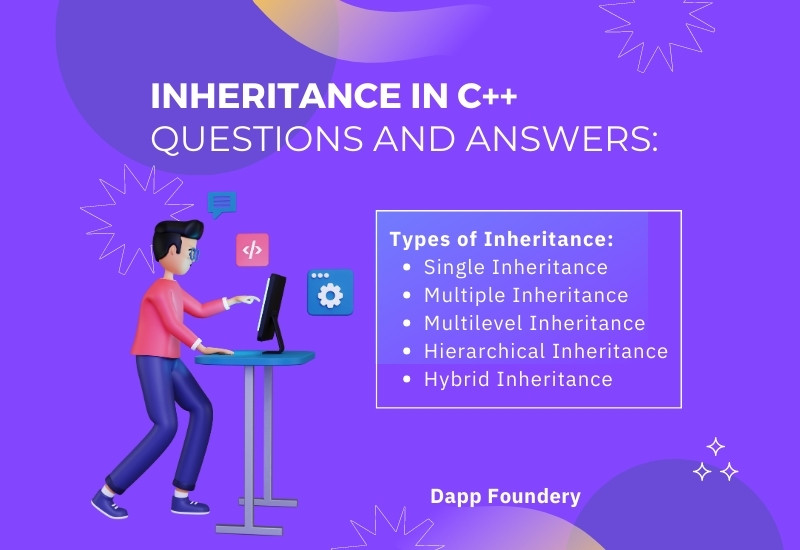
What is Inheritance in C++?
Inheritance is a fundamental concept in object-oriented programming (OOP) that allows a class to inherit properties and behaviors from another class. It enables code reusability, promotes modularity, and simplifies program development. In C++, inheritance forms an essential part of creating class hierarchies and implementing complex relationships between classes.
Benefits of Inheritance in C++:
Inheritance provides several advantages in C++. Some of the key benefits include:
- Code Reusability: Inheritance allows you to reuse existing code from base classes, saving time and effort in programming.
- Modularity: Inheritance promotes modularity by organizing classes into hierarchies and enabling independent development and maintenance of related classes.
- Polymorphism: Inheritance facilitates polymorphism, enabling objects of different derived classes to be treated as objects of a common base class.
- Overriding and Overloading: Inheritance supports method overriding and function overloading, allowing derived classes to modify or extend the behavior of base class methods.
Types of Inheritance in C++ :
n C++, there are several types of inheritance available, each serving specific purposes. Let's explore them briefly:
Single Inheritance:
Single inheritance involves a derived class inheriting from a single base class. It establishes a one-to-one relationship between classes, where the derived class extends the functionality of the base class.
Multiple Inheritance:
Multiple inheritance allows a derived class to inherit from multiple base classes. This type of inheritance facilitates combining features and behaviors from different classes into a single derived class.
Multilevel Inheritance:
Multilevel inheritance involves a derived class inheriting from another derived class. It forms a hierarchy of classes, where each derived class adds new features and behaviors.
Hierarchical Inheritance:
Hierarchical inheritance occurs when multiple derived classes inherit from a single base class. It establishes a one-to-many relationship, where multiple classes extend the functionality of the same base class.
Hybrid Inheritance:
Hybrid inheritance is a combination of multiple inheritance and hierarchical inheritance. It allows a derived class to inherit from multiple base classes, including a mix of single and multiple inheritance.
Polymorphism and Inheritance:
Polymorphism is a powerful feature of inheritance in C++. It allows objects of different derived classes to be treated as objects of a common base class. Polymorphism enables dynamic binding, where the appropriate function or method is called based on the actual type of the object at runtime. This flexibility and extensibility make C++ inheritance a valuable tool in creating robust and flexible code structures.
Virtual Functions in Inheritance:
Virtual functions play a vital role in achieving polymorphism in C++ inheritance. A virtual function is a function declared in a base class that can be overridden by derived classes. When a derived class overrides a virtual function, the derived class's version of the function is called instead of the base class's version. Virtual functions enable dynamic binding, allowing the appropriate function to be called based on the object's actual type.
Constructors and Destructors in Inheritance:
Constructors and destructors are also inherited in C++ inheritance. When a derived class is created, the base class's constructor is called first, followed by the derived class's constructor. Similarly, when an object is destroyed, the derived class's destructor is called first, followed by the base class's destructor. This ensures proper initialization and cleanup of inherited members.
Overriding and Overloading in Inheritance:
In C++ inheritance, overriding and overloading are essential concepts. Overriding refers to the ability of a derived class to provide its implementation of a base class's virtual function. The overridden function in the derived class should have the same signature as the base class's virtual function. Overloading, on the other hand, allows the derived class to define multiple functions with the same name but different parameters, giving it added flexibility in handling different cases.
Inheritance in C++ Questions and Answers :
What is inheritance, and why is it important in C++?
- Explain the difference between single inheritance and multiple inheritance.
- Single inheritance involves a derived class inheriting from a single base class, establishing a one-to-one relationship. Multiple inheritance allows a derived class to inherit from multiple base classes, combining features and behaviors from different classes into a single derived class.
- How are constructors and destructors inherited in C++ inheritance?
- Constructors and destructors are inherited in C++ inheritance. When a derived class is created, the base class's constructor is called first, followed by the derived class's constructor. Similarly, when an object is destroyed, the derived class's destructor is called first, followed by the base class's destructor.
- What are virtual functions and why are they used in inheritance?
- Virtual functions are functions declared in a base class that can be overridden by derived classes. They are used in inheritance to achieve polymorphism, allowing objects of different derived classes to be treated as objects of a common base class. Virtual functions enable dynamic binding, where the appropriate function is called based on the object's actual type at runtime.
- Discuss the concept of function overriding in C++ inheritance.
- Function overriding occurs when a derived class provides its implementation of a base class's virtual function. The overridden function in the derived class should have the same signature as the base class's virtual function. When a function is called on an object of the derived class, the derived class's version of the function is executed instead of the base class's version.
- When would you choose composition over inheritance?
- Composition is preferred over inheritance when the relationship between classes is not a "is-a" relationship, but rather a "has-a" relationship. Composition involves creating objects of other classes within a class to utilize their functionalities. It offers more flexibility and avoids the potential drawbacks of deep class hierarchies associated with inheritance.
- What is the diamond problem in multiple inheritance, and how can it be resolved?
- The diamond problem occurs in multiple inheritance when a class inherits from two or more classes that have a common base class. It leads to ambiguity in accessing the common base class's members. The diamond problem can be resolved by using virtual inheritance. By declaring the base class as virtual during inheritance, it ensures that only a single instance of the base class is inherited.
- Can a derived class access the private members of its base class?
- No, a derived class cannot directly access the private members of its base class. Private members are only accessible within the class that declares them. However, the derived class can access the base class's private members through public or protected member functions or by making the base class a friend of the derived class.
Conclusion
Inheritance is a fundamental concept in C++ that allows classes to inherit properties and behaviors from other classes, promoting code reusability, modularity, and polymorphism. By understanding the types of inheritance, virtual functions, and guidelines for usage, you can leverage the power of inheritance to create well-structured and flexible code.